mirror of
https://github.com/redhat-actions/podman-login.git
synced 2025-02-23 18:41:21 +01:00
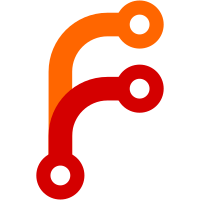
* Set env var REGISTRY_AUTH_FILE to work with buildah Signed-off-by: divyansh42 <diagrawa@redhat.com> * Add info level message Signed-off-by: divyansh42 <diagrawa@redhat.com> * Resolve reviews Signed-off-by: divyansh42 <diagrawa@redhat.com> * Reslve reviews Signed-off-by: divyansh42 <diagrawa@redhat.com>
70 lines
2.1 KiB
TypeScript
70 lines
2.1 KiB
TypeScript
/***************************************************************************************************
|
|
* Copyright (c) Red Hat, Inc. All rights reserved.
|
|
* Licensed under the MIT License. See LICENSE file in the project root for license information.
|
|
**************************************************************************************************/
|
|
|
|
import * as core from "@actions/core";
|
|
import * as io from "@actions/io";
|
|
import * as os from "os";
|
|
import * as path from "path";
|
|
import { getInputs } from "./context";
|
|
import { execute } from "./utils";
|
|
import * as stateHelper from "./state-helper";
|
|
|
|
let podmanPath: string | undefined;
|
|
|
|
async function getPodmanPath(): Promise<string> {
|
|
if (podmanPath == null) {
|
|
podmanPath = await io.which("podman", true);
|
|
await execute(podmanPath, [ "version" ], { group: true });
|
|
}
|
|
|
|
return podmanPath;
|
|
}
|
|
|
|
async function run(): Promise<void> {
|
|
if (os.platform() !== "linux") {
|
|
throw new Error("❌ Only supported on linux platform");
|
|
}
|
|
|
|
const {
|
|
registry, username, password, logout,
|
|
} = getInputs();
|
|
|
|
stateHelper.setRegistry(registry);
|
|
stateHelper.setLogout(logout);
|
|
|
|
const args = [
|
|
"login",
|
|
registry,
|
|
"-u",
|
|
username,
|
|
"-p",
|
|
password,
|
|
];
|
|
|
|
await execute(await getPodmanPath(), args);
|
|
core.info(`✅ Successfully logged in to ${registry} as ${username}`);
|
|
|
|
// Setting REGISTRY_AUTH_FILE environment variable as buildah needs
|
|
// this environment variable to point to registry auth file
|
|
const podmanAuthFilePath = path.join("/", "tmp", `podman-run-${process.getuid()}`,
|
|
"containers", "auth.json");
|
|
const REGISTRY_AUTH_ENVVAR = "REGISTRY_AUTH_FILE";
|
|
core.info(`Exporting ${REGISTRY_AUTH_ENVVAR}=${podmanAuthFilePath}`);
|
|
core.exportVariable(REGISTRY_AUTH_ENVVAR, podmanAuthFilePath);
|
|
}
|
|
|
|
async function registryLogout(): Promise<void> {
|
|
if (!stateHelper.logout) {
|
|
return;
|
|
}
|
|
await execute(await getPodmanPath(), [ "logout", stateHelper.registry ]);
|
|
}
|
|
|
|
if (!stateHelper.IsPost) {
|
|
run().catch(core.setFailed);
|
|
}
|
|
else {
|
|
registryLogout().catch(core.setFailed);
|
|
}
|